Python: Overload decorator
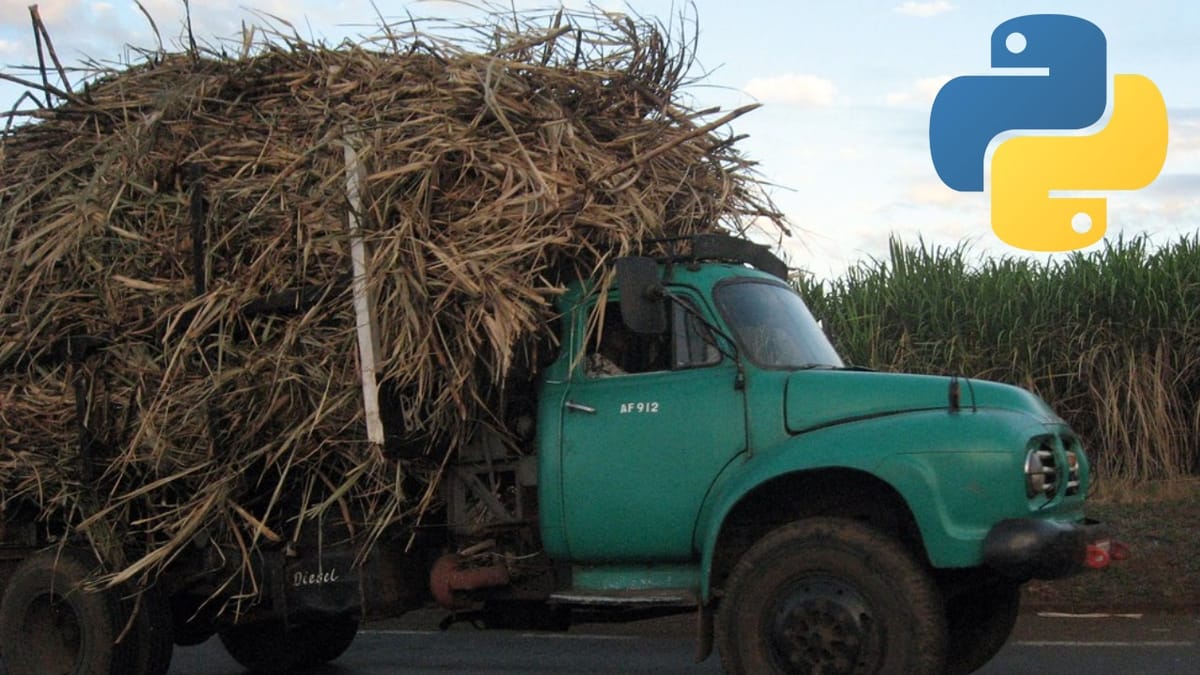
Type annotations were introduced in Python with PEP 484 and were first made available in Python 3.5 around 2015. Type annotations provide a way for code to be explicitly annotated with types, allowing static type checkers, IDEs, and other tools to more effectively understand the code. While these annotations are available for use, it's important to note that they do not enforce type checking at runtime unless specifically checked using third-party libraries like mypy
.
Adding an @overload
function declaration helps type checkers by providing explicit information about the different ways a function can be called and what types it can accept or return, which might not be apparent from the implementation signature alone. The implementation signature, especially in dynamically typed languages like Python, is often more generic and may not clearly convey all the nuances of how a function is supposed to be used with different types of arguments. Let's explore some of the reasons why overloading is beneficial for type checking:
1. Multiple Signatures for a Single Function
A function might be designed to accept different types of arguments and return different types based on those inputs. By using @overload
, you can declare each of these signatures explicitly. This level of detail helps type checkers understand exactly which argument types are valid and what the corresponding return type will be, enhancing the accuracy of type checking.
2. Complex Type Logic
Some functions perform internal type checking and behave differently based on the types of the provided arguments. The implementation might use a single, broad type that encompasses multiple specific types (e.g., using Union
), making it unclear which combinations of argument types are intended to work together. Overloads allow developers to specify these combinations explicitly.
3. Keyword Arguments and Defaults
Functions in Python can have keyword arguments with default values, leading to different behaviors depending on whether those keywords are provided. Overloaded signatures can specify which combinations of arguments are valid, including with or without certain keyword arguments, providing clearer guidance to the type checker.
4. Enhanced Readability and Documentation
Overloads also serve as documentation, making it easier for developers to understand the intended uses of a function. This is especially useful in large code bases or libraries where functions may have complex logic catering to different scenarios.
Example
Consider a simplified example of a function that can take either a string or a list of strings:
from typing import List, Union, overload
# Overloaded declarations
@overload
def process(items: List[str]) -> List[str]: ...
@overload
def process(items: str) -> str: ...
# Implementation
def process(items: Union[List[str], str]) -> Union[List[str], str]:
if isinstance(items, list):
return [item.upper() for item in items]
return items.upper()
The process function responds differently depending on the argument type. By explicitly listing the possible function signatures for the process
function using the @overload
decorator, you can achieve the benefits listed above.
Comments ()